|
字符串是一种非常重要的数据类型,但是C语言不存在显式的字符串类型,C语言中的字符串都以字符串常量的形式出现或存储在字符数组中。同时,C 语言提供了一系列库函数来对操作字符串,这些库函数都包含在头文件 string.h 中。
1.strtok 实现字符串切割: 将字符串根据分隔符进行切割分片.
#include <stdio.h>
int main(int argc, char* argv[])
{
char str[] = &#34;hello,lyshark,welcome&#34;;
char *ptr;
ptr = strtok(str, &#34;,&#34;);
while (ptr != NULL)
{
printf(&#34;切割元素: %s\n&#34;, ptr);
ptr = strtok(NULL, &#34;,&#34;);
}
system(&#34;pause&#34;);
return 0;
}2.strlen 获取字符串长度
#include <stdio.h>
int main(int argc, char* argv[])
{
char Array[] = &#34;\0hello\nlyshark&#34;;
char Str[] = { &#39;h&#39;, &#39;e&#39;, &#39;l&#39;, &#39;l&#39;, &#39;o&#39; };
int array_len = strlen(Array);
printf(&#34;字符串的有效长度:%d\n&#34;, array_len);
int str_len = strlen(Str);
printf(&#34;字符串数组有效长度: %d\n&#34;, str_len);
int index = 0;
while (Str[index] != &#39;\0&#39;)
{
index++;
printf(&#34;Str数组元素: %c --> 计数: %d \n&#34;, Str[index], index);
}
system(&#34;pause&#34;);
return 0;
}3.strcpy 字符串拷贝:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, char* argv[])
{
char Array[] = &#34;hello lyshark&#34;;
char tmp[100];
// 学习strcpy函数的使用方式
if (strcpy(tmp, Array) == NULL)
printf(&#34;从Array拷贝到tmp失败\n&#34;);
else
printf(&#34;拷贝后打印: %s\n&#34;, tmp);
// 清空tmp数组的两种方式
for (unsigned int x = 0; x < strlen(tmp); x++)
tmp[x] = &#39; &#39;;
memset(tmp, 0, sizeof(tmp));
// 学习strncpy函数的使用方式
if (strncpy(tmp, Array, 3) == NULL)
printf(&#34;从Array拷贝3个字符到tmp失败\n&#34;);
else
printf(&#34;拷贝后打印: %s\n&#34;, tmp);
system(&#34;pause&#34;);
return 0;
}4.strcat字符串连接: 将由src指向的空终止字节串的副本追加到由dest指向的以空字节终止的字节串的末尾
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, char* argv[])
{
char str1[50] = &#34;hello &#34;;
char str2[50] = &#34;lyshark!&#34;;
char * str = strcat(str1, str2);
printf(&#34;字符串连接: %s \n&#34;, str);
str = strcat(str1, &#34; world&#34;);
printf(&#34;字符串连接: %s \n&#34;, str);
str = strncat(str1, str2, 3);
printf(&#34;字符串连接: %s \n&#34;, str);
system(&#34;pause&#34;);
return 0;
}5.strcmp 字符串对比:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int Str_Cmp(const char * lhs, const char * rhs)
{
int ret = strcmp(lhs, rhs);
if (ret == 0)
return 1;
else
return 0;
}
int main(int argc, char* argv[])
{
char *str1 = &#34;hello lyshark&#34;;
char *str2 = &#34;hello lyshark&#34;;
int ret = Str_Cmp(str1, str2);
printf(&#34;字符串是否相等: %d \n&#34;, ret);
if (!strncmp(str1, str2, 3))
printf(&#34;两个字符串,前三位相等&#34;);
system(&#34;pause&#34;);
return 0;
}6.strshr 字符串截取:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, char* argv[])
{
const char str[] = &#34;hello ! lyshark&#34;;
char *ret;
ret = strchr(str, &#39;!&#39;);
printf(&#34;%s \n&#34;, ret);
system(&#34;pause&#34;);
return 0;
}7.字符串逆序排列:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
void Swap_Str(char *Array)
{
int len = strlen(Array);
char *p1 = Array;
char *p2 = &Array[len - 1];
while (p1 < p2)
{
char tmp = *p1;
*p1 = *p2;
*p2 = tmp;
p1++, p2--;
}
}
int main(int argc, char* argv[])
{
char str[20] = &#34;hello lyshark&#34;;
Swap_Str(str);
for (int x = 0; x < strlen(str); x++)
printf(&#34;%c&#34;, str[x]);
system(&#34;pause&#34;);
return 0;
}8.实现字符串拷贝:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
// 使用数组实现字符串拷贝
void CopyString(char *dest,const char *source)
{
int len = strlen(source);
for (int x = 0; x < len; x++)
{
dest[x] = source[x];
}
dest[len] = &#39;\0&#39;;
}
// 使用指针的方式实现拷贝
void CopyStringPtr(char *dest, const char *source)
{
while (*source != &#39;\0&#39;)
{
*dest = *source;
++dest, ++source;
}
*dest = &#39;\0&#39;;
}
// 简易版字符串拷贝
void CopyStringPtrBase(char *dest, const char *source)
{
while (*dest++ = *source++);
}
int main(int argc, char* argv[])
{
char * str = &#34;hello lyshark&#34;;
char buf[1024] = { 0 };
CopyStringPtrBase(buf, str);
printf(&#34;%s \n&#34;, buf);
system(&#34;pause&#34;);
return 0;
}9.格式化字符串:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, char* argv[])
{
// 格式化填充输出
char buf[30] = { 0 };
sprintf(buf, &#34;hello %s %s&#34;, &#34;lyshark&#34;,&#34;you are good&#34;);
printf(&#34;格式化后: %s \n&#34;, buf);
// 拼接字符串
char *s1 = &#34;hello&#34;;
char *s2 = &#34;lyshark&#34;;
memset(buf, 0, 30);
sprintf(buf, &#34;%s --> %s&#34;, s1, s2);
printf(&#34;格式化后: %s \n&#34;, buf);
// 数字装换位字符串
int number = 100;
memset(buf, 0, 30);
sprintf(buf, &#34;%d&#34;, number);
printf(&#34;格式化后: %s \n&#34;, buf);
system(&#34;pause&#34;);
return 0;
}10.动态存储字符串:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main(int argc, char* argv[])
{
// 分配空间
char **p = malloc(sizeof(char *)* 5);
for (int x = 0; x < 5;++x)
{
p[x] = malloc(64);
memset(p[x], 0, 64);
sprintf(p[x], &#34;Name %d&#34;, x + 1);
}
// 打印字符串
for (int x = 0; x < 5; x++)
printf(&#34;%s \n&#34;, p[x]);
// 释放空间
for (int x = 0; x < 5; x++)
{
if (p[x] != NULL)
free(p[x]);
}
system(&#34;pause&#34;);
return 0;
}11.字符串拼接:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char * StringSplicing(char *String1, char *String2)
{
char Buffer[1024];
int index = 0;
int len = strlen(String1);
while (String1[index] != &#39;\0&#39;)
{
Buffer[index] = String1[index];
index++;
}
while (String2[index - len] != &#39;\0&#39;)
{
Buffer[index] = String2[index - len];
index++;
}
Buffer[index] = &#39;\0&#39;;
char *ret = (char*)calloc(1024, sizeof(char*));
if (ret)
strcpy(ret, Buffer);
return ret;
}
int main(int argc, char* argv[])
{
char *str1 = &#34;hello &#34;;
char *str2 = &#34;lyshark ! \n&#34;;
char * new_str = StringSplicing(str1, str2);
printf(&#34;拼接好的字符串是: %s&#34;, new_str);
system(&#34;pause&#34;);
return 0;
}12.实现strchr:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char * MyStrchr(const char *String, char ch)
{
char *ptr = String;
while (*ptr != &#39;\0&#39;)
{
if (*ptr == ch)
return ptr;
ptr++;
}
return NULL;
}
int main(int argc, char* argv[])
{
char Str[] = &#34;hello lyshark&#34;;
char ch = &#39;s&#39;;
char *ptr = MyStrchr(Str, ch);
printf(&#34;输出结果: %s \n&#34;, ptr);
system(&#34;pause&#34;);
return 0;
}13.自己实现寻找字符串子串:
#include <stdio.h>
#include <stdlib.h>
// 查找子串第一次出现的位置
char *MyStrStr(const char* str, const char* substr)
{
const char *mystr = str;
const char *mysub = substr;
while (*mystr != &#39;\0&#39;)
{
if (*mystr != *mysub)
{
++mystr;
continue;
}
char *tmp_mystr = mystr;
char *tmp_mysub = mysub;
while (tmp_mysub != &#39;\0&#39;)
{
if (*tmp_mystr != *tmp_mysub)
{
++mystr;
break;
}
++tmp_mysub;
}
if (*tmp_mysub == &#39;\0&#39;)
{
return mystr;
}
}
return NULL;
}
int main(int argc, char* argv[])
{
char *str = &#34;abcdefg&#34;;
char *sub = &#34;fg&#34;;
char * aaa = MyStrStr(str, sub);
printf(&#34;%s&#34;, aaa);
system(&#34;pause&#34;);
return 0;
}14.删除字符串中连续字符
#include <stdio.h>
char del(char s[],int pos,int len) //自定义删除函数,这里采用覆盖方法
{
int i;
for (i=pos+len-1; s!=&#39;\0&#39;; i++,pos++)
s[pos-1]=s; //用删除部分后的字符依次从删除部分开始覆盖
s[pos-1]=&#39;\0&#39;;
return s;
}
int main(int argc, char *argv[])
{
char str[50];
int position,length;
printf (&#34;please input string:\n&#34;);
gets(str); //使用gets函数获得字符串
printf (&#34;please input delete position:&#34;);
scanf(&#34;%d&#34;,&position);
printf (&#34;please input delete length:&#34;);
scanf(&#34;%d&#34;,&length);
del(str,position,length);
printf (&#34;the final string:%s\n&#34;,str);
return 0;
}
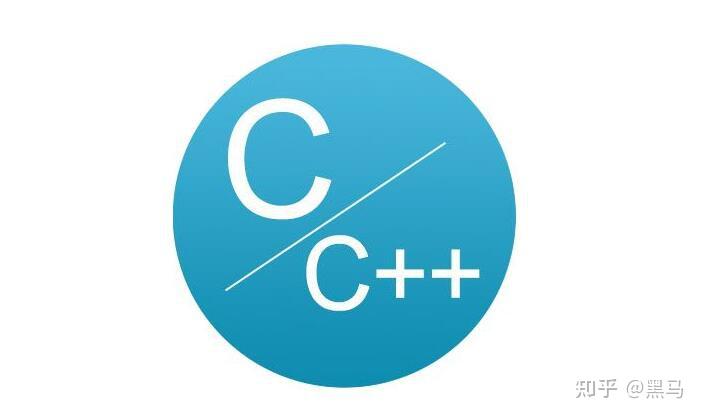
作者:程序猿小馆
链接:C语言字符串处理
出处:CSDN |
|